Create Fillable PDF Form
The Pdfcrowd HTML to PDF API makes it easy to programmatically create fillable PDF forms from standard HTML forms. The API supports most of the HTML form features and, together with support for digital signatures (see Create Digital Signature in PDF), provides an easy way to create PDF forms.
This feature is available starting with Pdfcrowd API client libraries version 5.6.
Simple Example
Add an HTML form field, for example a text input:
<p>Enter name: <input type="text"></p>
Enable generation of fillable PDF forms with the setEnablePdfForms() method:
client.setEnablePdfForms(true)
The resulting PDF will display an interactive input text field.
See: Example PDF, HTML and Code
Form with Buttons Example
The API supports the submit and reset form actions. The following example uses both button types. The submit button makes an HTTP POST request, the reset button resets the form to the defaults.
<!DOCTYPE html>
<html>
<body>
<h1>Agreement</h1>
<p>
... body of the contract ...
</p>
<form action="https://your-url/" method="post">
<p>
I
<input type="text" value="enter your name" name="signer">
agree with the contract
<input type="checkbox" name="agree">
</p>
<p>
<input type="reset">
<input type="submit" value="Send">
</p>
</form>
</body>
</html>
See: Example PDF, HTML and Code
Fancy Styling
If you want to apply fancy styling, such as a semi-transparent, gradient or image background, horizontal-only borders, and so on, do not style the input element directly, but enclose it in another element and style that element instead.
<!DOCTYPE html>
<html>
<head>
<title>Agreement - fancy styling</title>
<style>
input {
width: 100%;
height: 100%;
border: none;
background: none;
box-sizing: border-box;
}
.input-wrapper {
width: 2in;
height: 1in;
border-top: 2pt solid black;
border-bottom: 2pt solid black;
background:linear-gradient(135deg, #fcdf8a 0%,#f38381 100%);
}
.input-block {
background-color: #eee;
padding: 0.2rem 1rem 1rem 1rem;
}
</style>
</head>
<body>
<h1>Agreement</h1>
<p>
... body of the contract ...
</p>
<div class="input-block">
<div class="input-wrapper">
<input type="text">
</div>
</div>
</body>
</html>
See: Example PDF, HTML and Code
Add Fillable Form To Existing PDF
If you have a static PDF (scanned or with a static form), you can use the API to make it fillable. Use the original PDF as a multipage background and place an HTML form over it.
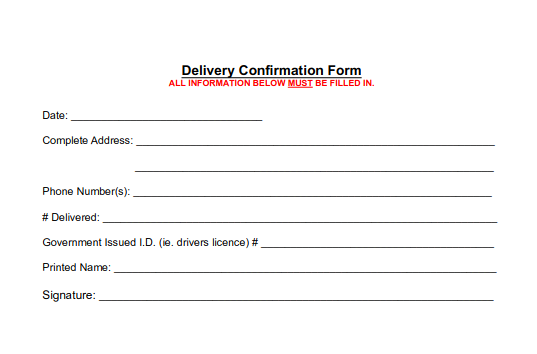
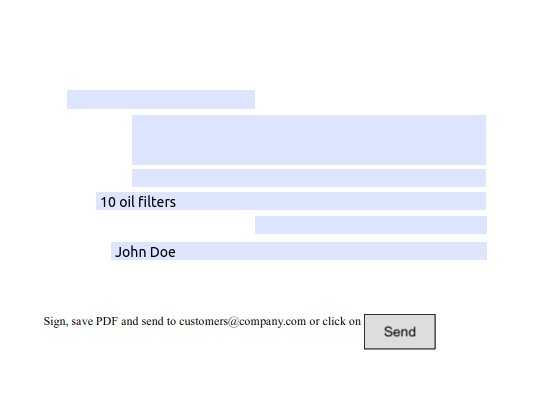
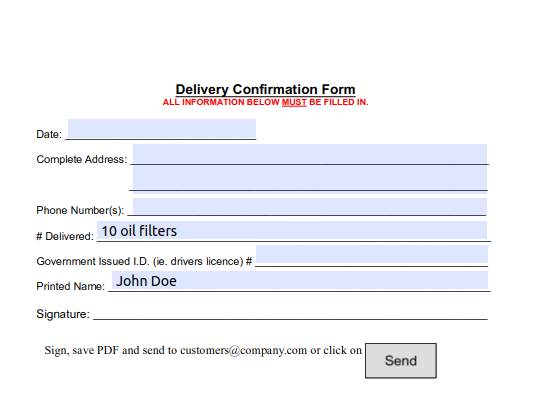
See: Background PDF, Output PDF, HTML and Code
Supported HTML Form Features
Pdfcrowd supports following HTML form elements:
- <input>
- <textarea>
- <select>
HTML form features supported:
- Multiple forms on a page
- Disabled, required and readonly attributes
- Selected and multiselect
- Min and max value for the number and range fields
- Maxlength for the text field
- Submit form data via an HTTP POST/GET request
- Reset form to default values
- Colors and borders
- Tooltips
PDF Specific Attributes
PDF features that are not available in HTML can be specified using custom HTML attributes:
data-pdfcrowd-font
By default, the font used for the HTML input field is embedded in the PDF file. To create a smaller PDF, you can specify a standard Adobe font using the data-pdfcrowd-font
attribute:
<input type="text" data-pdfcrowd-font="Courier">
You can use these standard Adobe fonts:
- Courier
- Courier Oblique
- Courier Bold
- Courier Bold-Oblique
- Helvetica
- Helvetica Oblique
- Helvetica Bold
- Helvetica Bold-Oblique
- Times Roman
- Times Italic
- Times Bold
- Times Bold-Italic
data-pdfcrowd-check-style
By default, the checkbox uses the check mark symbol and the radio uses the circle symbol. The data-pdfcrowd-check-style
attribute allows to specify a symbol from the Adobe Pi font. For example, for the circle symbol use:
<input type="checkbox" data-pdfcrowd-check-style="l">
Possible values:
- "l" - circle
- "4" - check
- "H" - star
- "u" - diamond
- "n" - rectangle
- "8" - cross
data-pdfcrowd-separate-thousands
Enables thousand separator.
<input type="number" data-pdfcrowd-separate-thousands>
data-pdfcrowd-decimal-places
Specifies the number of decimal places for the number input.
<input type="number" data-pdfcrowd-decimal-places="2">
data-pdfcrowd-negative-red
Displays a negative number in red.
<input type="number" data-pdfcrowd-negative-red>
data-pdfcrowd-parens
Encloses a negative number in parens.
<input type="number" data-pdfcrowd-parens>
data-pdfcrowd-symbol
Specifies a currency symbol for a number.
<input type="number" data-pdfcrowd-symbol="$">
data-pdfcrowd-prepend-symbol
Displays a currency symbol before a number.
<input type="number" data-pdfcrowd-symbol="$" data-pdfcrowd-prepend-symbol>
data-pdfcrowd-datetime-format
Specifies the format of the date input field.
<input type="date" data-pdfcrowd-datetime-format="m/d/yy HH:MM">
The format specifiers are:
- "m" - the month using one digit
- "mm" - the month using two digits
- "mmm" - the month using a short three characters
- "mmmm" - the month using full name
- "d" - the day using one digit
- "dd" - the day using two digits
- "ddd" - the day of the week using three characters
- "dddd" - the day of the week using full name
- "yy" - the year using two digits
- "yyyy" - the year using four digits
- "h" - the hour using one digit and 12 hours format
- "hh" - the hour using two digits and 12 hours format
- "H" - the hour using one digit and 24 hours format
- "HH" - the hour using two digits and 24 hours format
- "MM" - the number of minutes
- "s" - the number of seconds using one digit
- "ss" - the number of seconds using two digits
- "t" - the time relative to the meridiem - a or p
- "tt" - the time relative to the meridiem - am or pm
data-pdfcrowd-time-format
Specifies the format of the time input field.
<input type="time" data-pdfcrowd-time-format="HH:MM tt">
The format specifiers are:
- "h" - the hour using one digit and 12 hours format
- "hh" - the hour using two digits and 12 hours format
- "H" - the hour using one digit and 24 hours format
- "HH" - the hour using two digits and 24 hours format
- "MM" - the number of minutes
- "s" - the number of seconds using one digit
- "ss" - the number of seconds using two digits
- "t" - the time relative to the meridiem - a or p
- "tt" - the time relative to the meridiem - am or pm
data-pdfcrowd-submit-format
Specifies the format of the submitted form data.
<input type="submit" value="Submit FDF" data-pdfcrowd-submit-format="FDF">
The supported values are:
- HTML - HTML Form format, the default
- FDF - Forms Data Format
- XFDF - XML Forms Data Format
- PDF - the complete PDF
data-pdfcrowd-submit-canonical-dates
Submitted field values representing dates are converted to the standard format.
<input type="submit" data-pdfcrowd-submit-canonical-dates>
Exclude Input from Conversion
An input field with the data-pdfcrowd-static
attribute set is not interactive in the output PDF.
<input type="search" data-pdfcrowd-static>